swim.git
swim.h
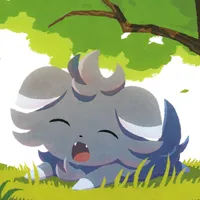
/* swim - window manager
* Copyright (C) 2022-2023 ArcNyxx
* see LICENCE file for licensing information */
#ifndef SWIM_H
#define SWIM_H
#include <stdbool.h>
#include <stdint.h>
#include <X11/Xlib.h>
#include <X11/extensions/Xrender.h>
#define BW 1
#define PW 14
#define H 18
enum { CC_PROTOCOLS, CC_DELETE, CC_STATE, CC_TAKE_FOCUS,
NET_NAME, NET_STATE, NET_FULL, NET_TYPE, NET_DIALOG,
NET_ACTIVE, NET_CLIENT_LIST, ATOM_LAST };
typedef enum clk { CLK_TAG, CLK_TITLE, CLK_STATUS, CLK_ROOT, CLK_CLI } clk_t;
typedef enum clr { CLR_NRM, CLR_SEL, CLR_TXT, CLR_LST } clr_t;
typedef struct but {
void (*func)(long);
long arg;
clk_t clk;
int mask, but;
} but_t;
typedef struct key {
void (*func)(long);
long arg;
int mod;
uint32_t keysym;
} keyp_t;
typedef struct cli {
char name[256];
struct mon *mon;
struct cli *next;
uint32_t win;
uint32_t tags;
uint16_t x, y, w, h;
bool flt : 1, fsc : 1, urg : 1, nfc : 1;
} cli_t;
typedef struct mon {
struct mon *next;
cli_t *cli, *sel;
uint32_t win, pic;
uint32_t tags;
uint16_t x, y, w, h;
uint8_t mfact, nmaster;
bool show : 1, gap : 1, top : 1;
} mon_t;
extern Display *dpy;
extern Atom atom[ATOM_LAST];
#endif /* SWIM_H */