swim.git
conv.c
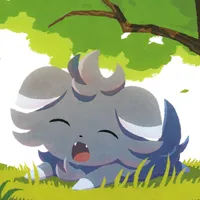
/* swim - window manager
* Copyright (C) 2022-2023 ArcNyxx
* see LICENCE file for licensing information */
#include <stdint.h>
#include <X11/Xlib.h>
#include "conv.h"
#include "swim.h"
#include "util.h"
extern mon_t *sel, *mons;
mon_t *
rectomon(uint16_t x, uint16_t y, uint16_t w, uint16_t h)
{
uint16_t area, last = 0;
mon_t *mon, *save = sel;
for (mon = mons; mon != NULL; mon = mon->next)
if ((area = MAX(0, MIN(x + w, mon->x + mon->w) -
MAX(x, mon->x)) *
MAX(0, MIN(y + h, mon->y + mon->h) -
MAX(y, mon->y))) > last)
last = area, save = mon;
return save;
}
cli_t *
wintocli(Window win)
{
for (mon_t *mon = mons; mon != NULL; mon = mon->next)
for (cli_t *cli = mon->cli; cli != NULL; cli = cli->next)
if (cli->win == win)
return cli;
return NULL;
}
mon_t *
wintomon(Window win)
{
int x, y;
if (win == ROOT && XQueryPointer(dpy, ROOT, &(Window){ 0 },
&(Window){ 0 }, &x, &y, &(int){ 0 }, &(int){ 0 },
&(unsigned int){ 0 }))
return rectomon(x, y, 1, 1);
for (mon_t *mon = mons; mon != NULL; mon = mon->next)
if (win == mon->win)
return mon;
cli_t *cli = wintocli(win);
return cli != NULL ? cli->mon : sel;
}