swim.git
bar.c
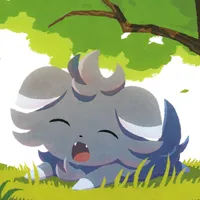
/* swim - window manager
* Copyright (C) 2022-2023 ArcNyxx
* see LICENCE file for licensing information */
#include <stdint.h>
#include "bar.h"
#include "draw.h"
#include "swim.h"
#include "util.h"
void
drawbar(const mon_t *mon)
{
extern int exec;
extern char stext[256], etext[256], *tags[9];
if (!mon->show)
return;
uint16_t sw = 0, w = 0, in;
if (stext[0] != '\0')
drawwide(stext, mon->w - PW, &sw), sw += PW, drawtext(mon,
CLR_NRM, CLR_TXT, mon->w - sw, sw);
uint32_t occ = 0, urg = 0;
for (cli_t *cli = mon->cli; cli != NULL; cli = cli->next)
occ |= cli->tags, urg |= cli->urg ? cli->tags : 0;
for (size_t i = 0; i < LENGTH(tags); ++i) {
in = 0, drawwide(tags[i], mon->w - w - PW, &in);
drawtext(mon, mon->tags & 1 << i ? CLR_SEL : CLR_NRM, CLR_TXT,
w, in + PW);
if (occ & 1 << i)
drawrect(mon, CLR_TXT, urg & 1 << i, w + 1, 1, 4, 4);
w += in + PW;
}
if ((sw = mon->w - sw - w) <= PW)
return;
if (exec == -1 && mon->sel == NULL)
drawrect(mon, CLR_NRM, true, w, 0, sw, H);
else
drawwide(exec != -1 ? etext : mon->sel->name, sw, &in),
drawtext(mon, CLR_SEL, CLR_TXT, w, sw);
}
void
drawbars(void)
{
extern mon_t *mons;
for (mon_t *mon = mons; mon != NULL; mon = mon->next)
drawbar(mon);
}