stego.git
table.py
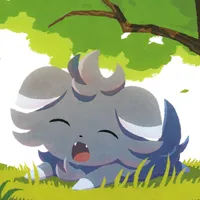
#!/bin/python3
# stego - chess engine
# Copyright (C) 2025 ArcNyxx <me@arcnyxx.net>
# see LICENCE file for licensing information
# use mailbox move generation to find possible move squares
mailbox = [
-1, -1, -1, -1, -1, -1, -1, -1, -1, -1,
-1, -1, -1, -1, -1, -1, -1, -1, -1, -1,
-1, 0, 1, 2, 3, 4, 5, 6, 7, -1,
-1, 8, 9, 10, 11, 12, 13, 14, 15, -1,
-1, 16, 17, 18, 19, 20, 21, 22, 23, -1,
-1, 24, 25, 26, 27, 28, 29, 30, 31, -1,
-1, 32, 33, 34, 35, 36, 37, 38, 39, -1,
-1, 40, 41, 42, 43, 44, 45, 46, 47, -1,
-1, 48, 49, 50, 51, 52, 53, 54, 55, -1,
-1, 56, 57, 58, 59, 60, 61, 62, 63, -1,
-1, -1, -1, -1, -1, -1, -1, -1, -1, -1,
-1, -1, -1, -1, -1, -1, -1, -1, -1, -1
]
mailbox64 = [
21, 22, 23, 24, 25, 26, 27, 28,
31, 32, 33, 34, 35, 36, 37, 38,
41, 42, 43, 44, 45, 46, 47, 48,
51, 52, 53, 54, 55, 56, 57, 58,
61, 62, 63, 64, 65, 66, 67, 68,
71, 72, 73, 74, 75, 76, 77, 78,
81, 82, 83, 84, 85, 86, 87, 88,
91, 92, 93, 94, 95, 96, 97, 98
]
def radial():
offsets = [ -10, -9, 1, 11, 10, 9, -1, -11 ]
directions = [ 'no', 'ne', 'ea', 'se', 'so', 'sw', 'we', 'nw' ]
rotab, bitab = [ 0 ] * 64, [ 0 ] * 64
for offset, direction in zip(offsets, directions):
di, dib = [], []
for index in mailbox64:
result = 0
move = index
while True:
move = mailbox[move + offset]
if move == -1:
break
result = result | (1 << move)
move = mailbox64[move]
di.append(result)
bit = 1 << 63 if offset > 0 else 1
dib.append(result | bit)
if abs(offset) in (1, 10):
rotab[mailbox[index]] = rotab[mailbox[index]] | result
else:
bitab[mailbox[index]] = bitab[mailbox[index]] | result
print(f'const u64 {direction}tab[64] = {{')
for dient in di:
print(f'\t{hex(dient)},')
print('};\n')
print(f'const u64 {direction}btab[64] = {{')
for dient in dib:
print(f'\t{hex(dient)},')
print('};\n')
print('const u64 rotab[64] = {')
for dient in rotab:
print(f'\t{hex(dient)},')
print('};\n')
print('const u64 bitab[64] = {')
for dient in bitab:
print(f'\t{hex(dient)},')
print('};\n')
def jump(name, offsets):
print(f'const u64 {name}tab[64] = {{')
for index in mailbox64:
result = 0
for offset in offsets:
move = mailbox[index + offset]
if move != -1:
result = result | (1 << move)
if name == 'wl':
result = result & 0xff00000000
elif name == 'bl':
result = result & 0xff000000
print(f'\t{hex(result)},')
print('};')
if name != 'bl':
print('')
print('/* stego - chess engine')
print(' * Copyright (C) 2025 ArcNyxx <me@arcnyxx.net>')
print(' * see LICENCE file for licensing information */\n')
print('#include "stego.h"\n')
jump('ki', [ -10, -9, 1, 11, 10, 9, -1, -11 ])
radial()
jump('kn', [ -21, -19, -12, -8, 8, 12, 19, 21 ])
jump('wc', [ -11, -9 ])
jump('bc', [ 9, 11 ])
jump('wp', [ -10 ])
jump('bp', [ 10 ])
jump('wl', [ -20 ])
jump('bl', [ 20 ])