stego.git
stego.h
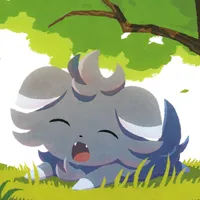
/* stego - chess engine
* Copyright (C) 2025 ArcNyxx <me@arcnyxx.net>
* see LICENCE file for licensing information */
#ifndef STEGO_H
#define STEGO_H
#include <stdbool.h>
#include <stdint.h>
typedef uint64_t u64;
typedef int sq_t;
typedef enum piece {
KING, QUEEN, ROOK, BISHOP, KNIGHT, PAWN, NONE
} piece_t;
typedef enum flag {
PQUEEN, PROOK, PBISHOP, PKNIGHT, NONE;
} flag_t;
typedef struct move {
sq_t from : 5, to : 5;
piece_t moved : 3;
flag_t flag : 3;
} move_t;
typedef struct tether {
u64 mask1, mask2;
piece_t piece1 : 3, piece2 : 3;
sq_t enpassant : 5;
bool wkcastle : 1, wqcastle : 1, bkcastle : 1, bqcastle : 1;
bool white1 : 1, white2 : 1;
} tether_t;
typedef struct board {
u64 wking, wqueen, wrook, wbishop, wknight, wpawn;
u64 bking, bqueen, brook, bbishop, bknight, bpawn;
u64 friend, enemy, occupied;
sq_t enpassant;
bool wkcastle : 1, wqcastle : 1, bkcastle : 1, bqcastle : 1;
bool white : 1;
} board_t;
#endif /* STEGO_H */