stego.git
perft.c
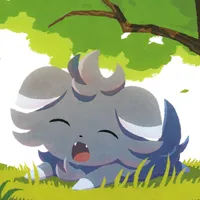
/* stego - chess engine
* Copyright (C) 2025 ArcNyxx <me@arcnyxx.net>
* see LICENCE file for licensing information */
#include <stdio.h>
#include <string.h>
#include "bits.h"
#include "move.h"
#include "stego.h"
u64
cnt(board_t *bd)
{
u64 ret = 0;
for (int i = 0; i < 64; ++i)
ret += popcount(bd->moves[i]);
return ret;
}
int
main(void)
{
board_t board, *bd = &board;
bd->king = bd->eking = 0b00010000;
bd->queen = bd->equeen = 0b00001000;
bd->rook = bd->erook = 0b10000001;
bd->bishop = bd->ebishop = 0b00100100;
bd->knight = bd->eknight = 0b01000010;
bd->pawn = bd->epawn = 0b11111111;
bd->epawn <<= 8, bd->pawn <<= 48;
bd->king <<= 56, bd->queen <<= 56, bd->rook <<= 56;
bd->bishop <<= 56, bd->knight <<= 56;
bd->enpassant = 64;
bd->wkcastle = bd->wqcastle = bd->bkcastle = bd->bqcastle = true;
bd->white = true;
memset(bd->moves, 0, sizeof(u64) * 64);
mv(bd);
printf("stego: first move: %llu\n", cnt(bd));
}