randpup.git
ngram.h
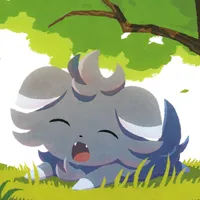
/* randpup - ngram text generator kernel module
* Copyright (C) 2025 ArcNyxx
* see LICENCE.MIT file for licensing information */
#ifndef PUP_NGRAM_H
#define PUP_NGRAM_H
#ifdef __KERNEL__
#include <linux/types.h>
#else /* __KERNEL__ */
#include <stdbool.h>
#endif /* __KERNEL__ */
#define STRING(str) { str, sizeof(str) - 1 }
/* 'next' is the index of an entry in the ngram table
* 'prob' is the probability score of a certain choice being selected, 'prob'
* numbers are iterated through linearly until less than or equal to a
* random number. they are sorted with the largest probability first to
* minimise the linear search time */
typedef struct pup_choice {
const int next, prob;
} pup_choice_t;
/* 'choice' is the starting index of the available entries in the choice table
* 'prob' is the maximum random number chosen for the linear search of the
* choice table using its 'prob' field
* 'ch' is the character printed when an ngram is selected */
typedef struct pup_ngram {
const int choice, prob;
const char ch;
} pup_ngram_t;
/* 'ngram' is the index of an entry in the ngram table
* 'prob' is the probability score of a certain starting ngram being selected,
* 'prob' numbers are iterated through linearly until less than or equal to
* a random number. they are sorted with the largest probability first to
* minimise the linear search time */
typedef struct pup_start {
const int ngram, prob;
const char ch;
} pup_start_t;
/* 'ngram' is the ngram table
* 'choice' is the choice table
* 'start' is the start table
* 'len' is the length of the ngram table
* 'prob' is the maximum random number chose for the linear search of the start
* table using its 'prob' field */
typedef struct pup_gen {
const pup_ngram_t *ngram;
const pup_choice_t *choice;
const pup_start_t *start;
const int len, prob;
} pup_gen_t;
typedef struct pup_string {
const char *str;
const int len;
} pup_string_t;
typedef struct pup_string_repeat {
const char *str;
const int len;
const int min, max;
const char ch;
} pup_string_repeat_t;
typedef struct pup_gendef {
const pup_gen_t *gen;
const int min, max;
} pup_gendef_t;
#endif /* PUP_NGRAM_H */