randpup.git
act.h
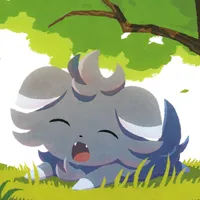
/* randpup - ngram text generator kernel module
* Copyright (C) 2025 ArcNyxx
* see LICENCE.MIT file for licensing information */
#ifndef PUP_ACT_H
#define PUP_ACT_H
#ifdef __KERNEL__
#include <linux/types.h>
#else /* __KERNEL__ */
#include <stdbool.h>
#endif /* __KERNEL__ */
#include "ngram.h"
#define MAX_ACT 3
typedef enum pup_type {
PUP_ACT_STRING, PUP_ACT_REPEAT, PUP_ACT_NGRAM
} pup_type_t;
typedef struct pup_ngram_act {
const pup_gen_t *gen;
int ngram, left;
bool start;
} pup_ngram_act_t;
typedef union pup_act {
pup_ngram_act_t ngram;
struct {
const char *str;
int ind, len;
};
struct {
char ch;
int left;
};
} pup_act_t;
typedef struct pup_state {
pup_act_t act[MAX_ACT];
pup_type_t type[MAX_ACT];
int ind, len, last;
} pup_state_t;
void pup_reset(pup_state_t *st);
ssize_t pup_write(pup_state_t *st, char *buf, size_t len);
#endif /* PUP_ACT_H */